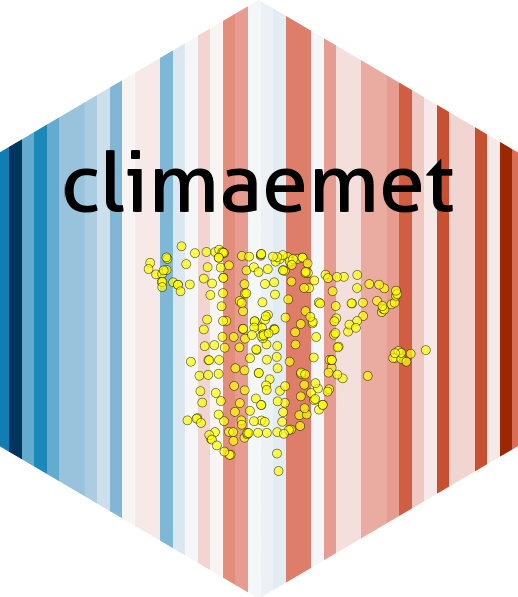
Forecast database by municipality
Source:R/aemet_forecast_daily.R
, R/aemet_forecast_hourly.R
aemet_forecast.Rd
Get a database of daily or hourly weather forecasts for a given municipality.
Usage
aemet_forecast_daily(
x,
verbose = FALSE,
extract_metadata = FALSE,
progress = TRUE
)
aemet_forecast_hourly(
x,
verbose = FALSE,
extract_metadata = FALSE,
progress = TRUE
)
Arguments
- x
A vector of municipality codes to extract. For convenience, climaemet provides this data on the dataset aemet_munic (see
municipio
field) as of January 2024.- verbose
Logical
TRUE/FALSE
. Provides information about the flow of information between the client and server.- extract_metadata
Logical
TRUE/FALSE
. OnTRUE
the output is atibble
with the description of the fields. See alsoget_metadata_aemet()
.- progress
Logical, display a
cli::cli_progress_bar()
object. Ifverbose = TRUE
won't be displayed.
Value
A nested tibble
. Forecasted values can be
extracted with aemet_forecast_tidy()
. See also Details.
Details
Forecasts format provided by the AEMET API have a complex structure.
Although climaemet returns a tibble
, each
forecasted value is provided as a nested tibble
.
aemet_forecast_tidy()
helper function can unnest these values an provide a
single unnested tibble
for the requested variable.
If extract_metadata = TRUE
a simple tibble
describing
the value of each field of the forecast is returned.
API Key
You need to set your API Key globally using aemet_api_key()
.
See also
aemet_munic for municipality codes and mapSpain package for
working with sf
objects of municipalities (see
mapSpain::esp_get_munic()
and Examples).
Other aemet_api_data:
aemet_alert_zones()
,
aemet_alerts()
,
aemet_beaches()
,
aemet_daily_clim()
,
aemet_extremes_clim()
,
aemet_forecast_beaches()
,
aemet_forecast_fires()
,
aemet_last_obs()
,
aemet_monthly
,
aemet_normal
,
aemet_stations()
Other forecasts:
aemet_forecast_beaches()
,
aemet_forecast_fires()
,
aemet_forecast_tidy()
Examples
# Select a city
data("aemet_munic")
library(dplyr)
munis <- aemet_munic %>%
filter(municipio_nombre %in% c("Santiago de Compostela", "Lugo")) %>%
pull(municipio)
daily <- aemet_forecast_daily(munis)
# Metadata
meta <- aemet_forecast_daily(munis, extract_metadata = TRUE)
glimpse(meta$campos)
#> Rows: 23
#> Columns: 5
#> $ id <chr> "id", "version", "elaborado", "nombre", "provincia", "fech…
#> $ descripcion <chr> "Indicativo de municipio", "Versión", "Fecha de elaboració…
#> $ tipo_datos <chr> "string", "float", "dataTime", "string", "string", "date",…
#> $ requerido <lgl> TRUE, TRUE, TRUE, TRUE, TRUE, FALSE, FALSE, FALSE, FALSE, …
#> $ unidad <chr> NA, NA, NA, NA, NA, NA, "Tanto por ciento (%)", "metros (m…
# Vars available
aemet_forecast_vars_available(daily)
#> [1] "probPrecipitacion" "cotaNieveProv" "estadoCielo"
#> [4] "viento" "rachaMax" "temperatura"
#> [7] "sensTermica" "humedadRelativa"
# This is nested
daily %>%
select(municipio, fecha, nombre, temperatura)
#> # A tibble: 14 × 4
#> municipio fecha nombre temperatura$maxima $minima $dato
#> <chr> <date> <chr> <int> <int> <list>
#> 1 15078 2025-02-10 Santiago de Compostela 16 8 <df>
#> 2 15078 2025-02-11 Santiago de Compostela 13 7 <df>
#> 3 15078 2025-02-12 Santiago de Compostela 15 6 <df>
#> 4 15078 2025-02-13 Santiago de Compostela 15 11 <df>
#> 5 15078 2025-02-14 Santiago de Compostela 17 10 <df>
#> 6 15078 2025-02-15 Santiago de Compostela 17 9 <df>
#> 7 15078 2025-02-16 Santiago de Compostela 17 6 <df>
#> 8 27028 2025-02-10 Lugo 14 6 <df>
#> 9 27028 2025-02-11 Lugo 12 6 <df>
#> 10 27028 2025-02-12 Lugo 12 6 <df>
#> 11 27028 2025-02-13 Lugo 13 6 <df>
#> 12 27028 2025-02-14 Lugo 15 7 <df>
#> 13 27028 2025-02-15 Lugo 17 6 <df>
#> 14 27028 2025-02-16 Lugo 15 5 <df>
# Select and unnest
daily_temp <- aemet_forecast_tidy(daily, "temperatura")
# This is not
daily_temp
#> # A tibble: 14 × 14
#> elaborado municipio nombre provincia id version uvMax fecha
#> <dttm> <chr> <chr> <chr> <chr> <dbl> <int> <date>
#> 1 2025-02-10 09:12:11 15078 Santi… A Coruña 15078 1 2 2025-02-10
#> 2 2025-02-10 09:12:11 15078 Santi… A Coruña 15078 1 2 2025-02-11
#> 3 2025-02-10 09:12:11 15078 Santi… A Coruña 15078 1 2 2025-02-12
#> 4 2025-02-10 09:12:11 15078 Santi… A Coruña 15078 1 2 2025-02-13
#> 5 2025-02-10 09:12:11 15078 Santi… A Coruña 15078 1 2 2025-02-14
#> 6 2025-02-10 09:12:11 15078 Santi… A Coruña 15078 1 NA 2025-02-15
#> 7 2025-02-10 09:12:11 15078 Santi… A Coruña 15078 1 NA 2025-02-16
#> 8 2025-02-10 09:12:11 27028 Lugo Lugo 27028 1 2 2025-02-10
#> 9 2025-02-10 09:12:11 27028 Lugo Lugo 27028 1 2 2025-02-11
#> 10 2025-02-10 09:12:11 27028 Lugo Lugo 27028 1 2 2025-02-12
#> 11 2025-02-10 09:12:11 27028 Lugo Lugo 27028 1 2 2025-02-13
#> 12 2025-02-10 09:12:11 27028 Lugo Lugo 27028 1 2 2025-02-14
#> 13 2025-02-10 09:12:11 27028 Lugo Lugo 27028 1 NA 2025-02-15
#> 14 2025-02-10 09:12:11 27028 Lugo Lugo 27028 1 NA 2025-02-16
#> # ℹ 6 more variables: temperatura_maxima <int>, temperatura_minima <int>,
#> # temperatura_6 <int>, temperatura_12 <int>, temperatura_18 <int>,
#> # temperatura_24 <int>
# Wrangle and plot
daily_temp_end <- daily_temp %>%
select(
elaborado, fecha, municipio, nombre, temperatura_minima,
temperatura_maxima
) %>%
tidyr::pivot_longer(cols = contains("temperatura"))
# Plot
library(ggplot2)
ggplot(daily_temp_end) +
geom_line(aes(fecha, value, color = name)) +
facet_wrap(~nombre, ncol = 1) +
scale_color_manual(
values = c("red", "blue"),
labels = c("max", "min")
) +
scale_x_date(
labels = scales::label_date_short(),
breaks = "day"
) +
scale_y_continuous(
labels = scales::label_comma(suffix = "º")
) +
theme_minimal() +
labs(
x = "", y = "",
color = "",
title = "Forecast: 7-day temperature",
subtitle = paste(
"Forecast produced on",
format(daily_temp_end$elaborado[1], usetz = TRUE)
)
)
# Spatial with mapSpain
library(mapSpain)
library(sf)
#> Linking to GEOS 3.12.2, GDAL 3.9.3, PROJ 9.4.1; sf_use_s2() is TRUE
lugo_sf <- esp_get_munic(munic = "Lugo") %>%
select(LAU_CODE)
daily_temp_end_lugo_sf <- daily_temp_end %>%
filter(nombre == "Lugo" & name == "temperatura_maxima") %>%
# Join by LAU_CODE
left_join(lugo_sf, by = c("municipio" = "LAU_CODE")) %>%
st_as_sf()
ggplot(daily_temp_end_lugo_sf) +
geom_sf(aes(fill = value)) +
facet_wrap(~fecha) +
scale_fill_gradientn(
colors = c("blue", "red"),
guide = guide_legend()
) +
labs(
main = "Forecast: 7-day max temperature",
subtitle = "Lugo, ES"
)